AI ML
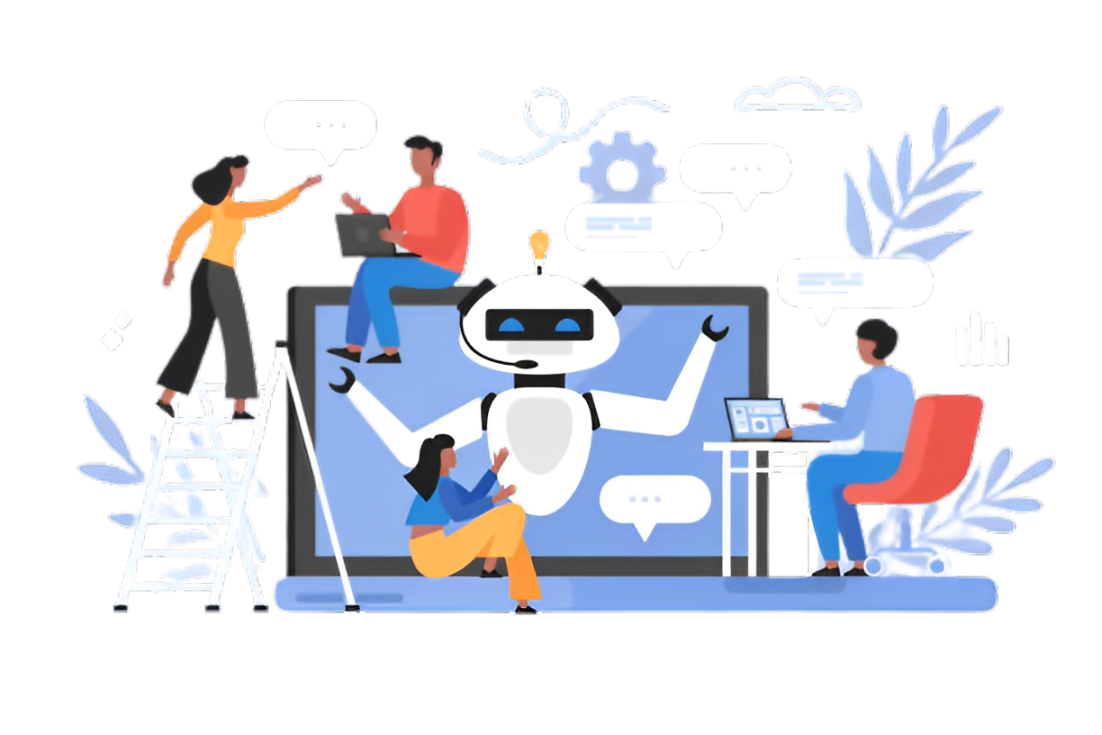
Master AI ML to turn data into insightful knowledge that will spur innovation and influence the future.
AI ML Course Duration: 45 Days
- 100% Practical
- Certification
- 7+ Project Assignments
- Agency Style Training
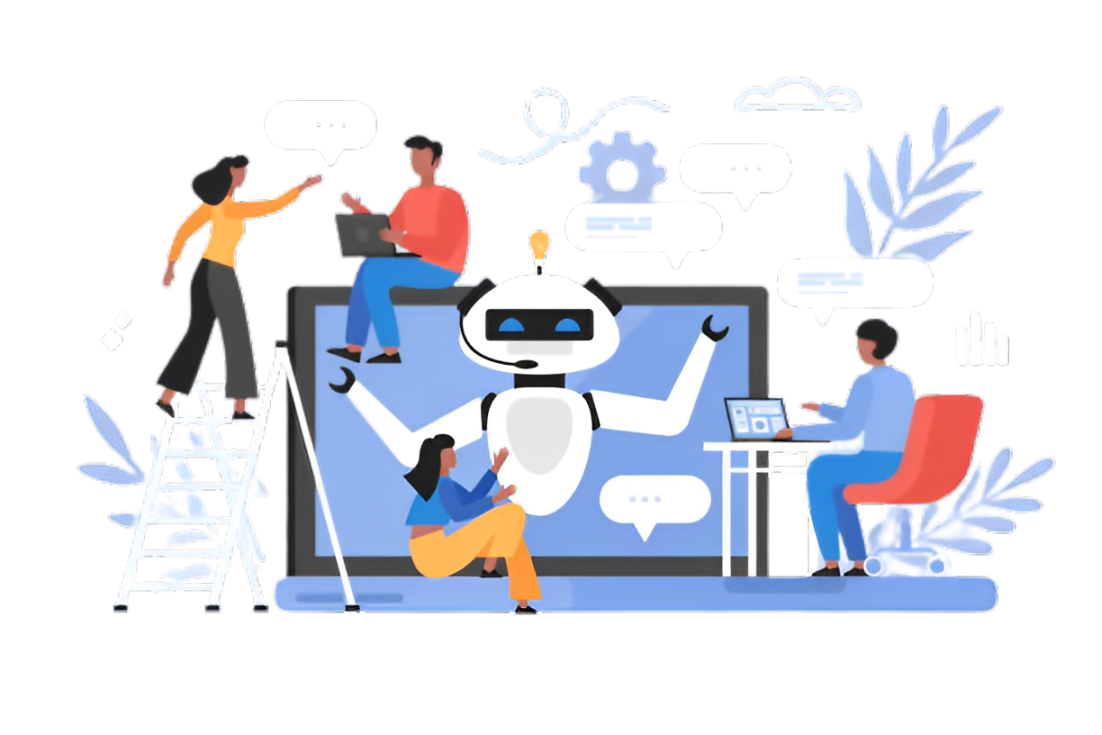
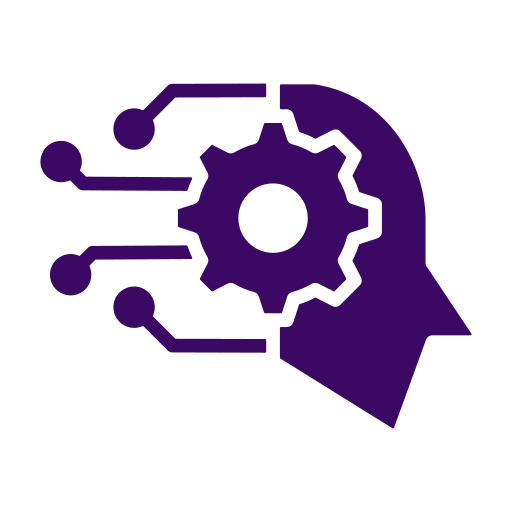
Real-World Applications
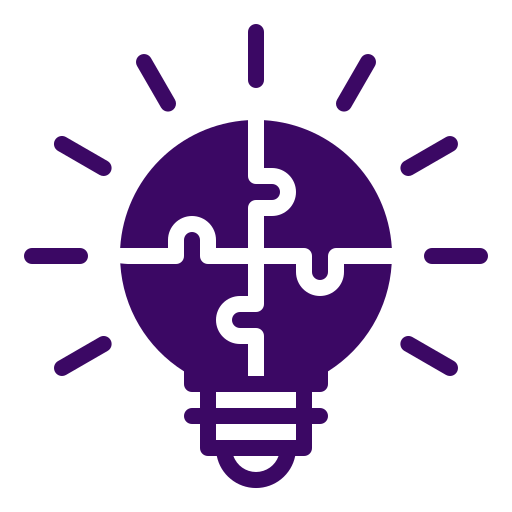
In-Demand Skills
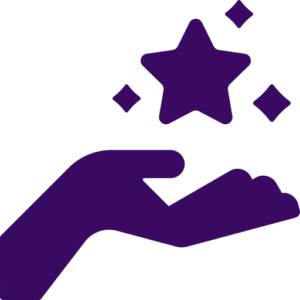
Expert Guidance
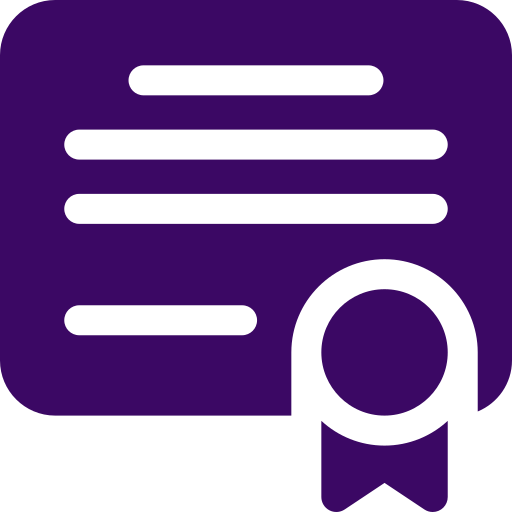
Certification
AI ML Learning Curriculum
- Getting Started with Python
1.1 Python Introduction and Environment Setup
- Introduction to Python programming language.
- Installing Python and setting up IDEs (e.g., PyCharm, VS Code, Jupyter Notebooks).
- Overview of Python’s applications in data science and machine learning.
1.2 Python Basic Syntax and Data Types
- Variables, constants, and comments.
- Data types: int, float, bool, string, and complex.
- Typecasting between different data types.
1.3 Operators in Python
- Arithmetic, comparison, logical, bitwise, assignment, and identity operators.
- Operator precedence and associativity.
1.4 Working with Strings
- Creating, indexing, slicing, and manipulating strings.
- String methods: split(), join(), replace(), upper(), lower(), etc.
1.5 Python Collections
- Lists: Creating, accessing, updating, and manipulating lists.
- Tuples: Characteristics of tuples, tuple operations, immutability.
- Sets: Operations on sets (union, intersection, difference), set methods.
- Dictionaries: Key-value pairs, dictionary methods, nested dictionaries.
1.6 Conditional Statements
- if, if-else, and if-elif-else statements.
- Nested conditions, and best practices for readability.
1.7 Loops in Python
- for and while loops.
- Using loop controls (break, continue, pass).
- Looping through collections and custom ranges.
1.8 List and Dictionary Comprehension
- Simplifying loops with list and dictionary comprehensions.
- Applications in data manipulation and generation.
1.9 Functions in Python
- Defining and calling functions.
- Arguments, keyword arguments, and default parameters.
- Returning values from functions.
1.10 Anonymous Functions (Lambda Expressions)
- Writing and using lambda functions.
- Using lambda with map(), filter(), and reduce().
1.11 Generators
- Introduction to generators and yield keyword.
- Differences between generators and iterators.
- Use cases for generators in memory-efficient programming.
1.12 Modules in Python
- Creating and importing modules.
- Using built-in modules (e.g., math, random, os).
- Working with third-party libraries (pip and package management).
1.13 Exceptions and Error Handling
- try, except, else, and finally blocks.
- Raising exceptions (raise), custom exceptions.
1.14 Object-Oriented Programming (OOP) in Python
- Classes and Objects: Creating classes, instantiating objects.
- Methods: Instance methods, class methods, static methods.
- Inheritance: Single, multiple inheritance, method overriding.
- Polymorphism: Method overloading and overriding.
- Operator Overloading: Overloading operators for custom object behavior.
1.15 Working with Dates and Times
- Introduction to datetime module.
- Parsing, formatting, and manipulating dates and times.
- Timezone handling and time arithmetic.
1.16 Regular Expressions (Regex)
- Introduction to regular expressions.
- Functions: re.search(), re.match(), re.compile(), re.split().
- Pattern matching, substitution, and validation.
1.17 File Handling
- Opening, reading, writing, and closing files in Python.
- Reading from and writing to CSV and JSON files.
- File pointers, modes (read, write, append), and exception handling in file operations.
1.18 APIs: The Unsung Hero of the Connected World
- Introduction to APIs, RESTful APIs.
- Using Python’s requests module to interact with APIs.
- Authentication, GET and POST requests, and handling API responses.
1.19 Python for Web Development: Flask
- Introduction to Flask web framework.
- Setting up a basic Flask project.
- Building web routes, rendering HTML templates.
- Connecting a Python app to a database (SQLite, MySQL).
1.20 Hands-On Python Projects
- Web Scraping: Using BeautifulSoup and requests to scrape data from websites.
- Sending Automated Emails: Using Python to send automated emails with attachments.
- Building a Virtual Assistant: Voice recognition, task automation, and response generation using Python.
Mathematics
2.1 Introduction to Statistics and Probability
- Descriptive statistics: mean, median, mode, variance, standard deviation.
- Probability concepts: conditional probability, Bayes’ theorem, probability distributions.
2.2 Linear Algebra
- Vectors, matrices, and matrix operations.
- Eigenvalues and eigenvectors.
- Dot product, cross product, and matrix factorization.
2.3 Calculus for Machine Learning
- Derivatives and integrals.
- Partial derivatives, gradients, and optimization.
Understanding cost functions and gradient descent.
Data Analysis and Visualization Libraries
3.1 Numpy
- Working with arrays, array operations, and broadcasting.
- Linear algebra with Numpy.
- Random number generation and simulations.
3.2 Pandas
- DataFrames, Series, and Indexes.
- Reading from and writing to CSV, Excel, and databases.
- Data manipulation: filtering, grouping, aggregating, merging, and reshaping.
3.3 Matplotlib and Seaborn
- Creating visualizations: line plots, scatter plots, bar charts, histograms.
- Customizing plots (titles, labels, legends).
- Using Seaborn for advanced plots (heatmaps, pair plots, and box plots).
3.4 Web Scraping
- Ethical considerations in web scraping.
- Tools and libraries: BeautifulSoup, Selenium, Scrapy.
- Storing and processing scraped data.
3.5 Exploratory Data Analysis (EDA)
- Techniques for exploring datasets.
- Identifying missing data, outliers, and data patterns.
- Visualizing distributions, correlations, and feature relationships.
3.6 Database Access and SQL
- SQL basics: SELECT, INSERT, UPDATE, DELETE.
- Joining tables, aggregations, and filtering data.
- Connecting Python to databases (e.g., SQLite, MySQL).
3.7 Power BI
- Introduction to Power BI desktop.
- Connecting to various data sources.
- Creating interactive dashboards and reports.
Data Manipulation and Preprocessing
4.1 Data Cleaning and Transformation
- Handling missing data: imputation, dropping.
- Dealing with duplicates, inconsistent data formats, and incorrect entries.
4.2 Feature Engineering
- Creating new features from existing data.
- Interaction terms, binning, encoding categorical variables (one-hot encoding).
4.3 Feature Scaling and Normalization
- Standardization vs normalization.
- Techniques: MinMaxScaler, StandardScaler.
4.4 Handling Categorical Variables
- Encoding categorical variables: LabelEncoder, OneHotEncoder.
- Dealing with high cardinality categorical variables.
Machine Learning
5.1 Supervised Learning
- Regression Models:
- Simple Linear Regression, Polynomial Regression, Ridge, Lasso Regression.
- Practical applications and implementation using scikit-learn.
- Classification Models:
- Logistic Regression, Decision Trees, Random Forests, Support Vector Machines (SVM).
- Performance metrics: Confusion matrix, ROC curve, AUC, Precision, Recall, F1-score.
5.2 Unsupervised Learning
- Clustering:
- K-Means Clustering, Hierarchical Clustering, DBSCAN.
- Visualizing clusters and analyzing cluster results.
- Dimensionality Reduction:
- Principal Component Analysis (PCA), t-SNE for data visualization.
5.3 Model Evaluation and Validation
- Train-test split, cross-validation.
- Hyperparameter tuning: GridSearchCV, RandomizedSearchCV.
Overfitting, underfitting, and model selection.
Deep Learning
6.1 Artificial Neural Networks (ANN)
- Structure of neural networks: neurons, layers, activation functions.
- Forward propagation, backpropagation, and gradient descent.
6.2 Convolutional Neural Networks (CNN)
- CNN architecture: Convolution layers, pooling layers, fully connected layers.
- Applications of CNNs: Image classification, object detection.
- Hands-on: Implementing CNNs using TensorFlow/Keras
6.3 Recurrent Neural Networks (RNN)
- Introduction to sequence data.
- Understanding vanishing gradients and the role of LSTMs and GRUs.
- Applications: Time-series forecasting, text generation.
6.4 Long Short-Term Memory (LSTM) Networks
- Understanding LSTM architecture.
- Use cases: Sentiment analysis, sequence prediction, speech recognition.
6.5 Generative Adversarial Networks (GANs)
- GAN architecture: Generator and discriminator networks.
- Training GANs: Understanding adversarial training and loss functions.
- Applications of GANs: Image generation, style transfer, synthetic data creation.
Natural Language Processing (NLP)
7.1 Text Preprocessing
- Tokenization, stopword removal, stemming, and lemmatization.
- Sentence splitting and named entity recognition (NER).
7.2 Text Representation
- Bag of Words, TF-IDF, and word embeddings.
- Word2Vec and GloVe embeddings for semantic analysis.
7.3 NLP Models
- Sentiment analysis, topic modeling, and text classification.
- Sequence-to-sequence models for language translation, summarization, and chatbot creation.
7.4 Transformer Models
- Introduction to transformer architectures.
- Understanding the attention mechanism.
- Pre-trained transformer models: BERT, GPT, T5.
- Fine-tuning transformer models for downstream tasks.
7.5 Large Language Models (LLMs)
- Overview of LLMs: GPT-3, GPT-4, BERT.
- Understanding zero-shot, few-shot, and fine-tuning capabilities of LLMs.
- Practical implementation: Text generation, summarization, and conversational AI.
Transfer Learning
8.1 Introduction to Transfer Learning
- Concept of transfer learning and pre-trained models.
- Applications in computer vision and NLP.
8.2 Pretrained Models for Image and Text
- Pretrained models in computer vision: VGG16, ResNet, Inception.
- Pretrained models in NLP: BERT, GPT, XLNet.
- Fine-tuning pretrained models for custom tasks.
8.3 Hands-On: Using Transfer Learning
- Implementing transfer learning in image classification using Keras/TensorFlow.
- Fine-tuning a pre-trained NLP model for text classification tasks.
Time Series
9.1 Introduction to Time Series Analysis
- Understanding time series data, decomposition into trend, seasonality, and noise.
9.2 Statistical Methods for Time Series
- Descriptive statistics: Mean, variance, autocorrelation.
- Smoothing techniques: Moving averages, exponential smoothing.
9.3 Stationarity and Transformation
- Testing for stationarity: ADF Test, KPSS Test.
- Differencing and log transformation.
9.4 ARIMA Models
- Building AR, MA, and ARIMA models for forecasting.
- Seasonal ARIMA (SARIMA) for handling seasonality.
9.5 Machine Learning Approaches
- Random Forest, Gradient Boosting for time series forecasting.
- Using RNN and LSTM for time series data.
9.6 Time Series Forecasting Libraries
- Python libraries for time series forecasting: statsmodels, Prophet, pmdarima.
9.7 Model Evaluation
- Forecast accuracy metrics: Mean Absolute Error (MAE), Mean Squared Error (MSE), Root Mean Squared Error (RMSE).
Advanced Deep Learning
10.1 Transfer Learning
- Using transfer learning for deep learning tasks in both computer vision and NLP.
10.2 Generative Adversarial Networks (GANs)
- Deep dive into advanced GAN architectures and applications.
10.3 NLP with Large Language Models (LLMs)
- Fine-tuning LLMs for custom tasks: text generation, summarization, translation.
10.4 Hands-On Projects
- Building real-world projects using Transfer Learning, GANs, and LLMs.
Project Work
11.1 Project Overview
- Objective: To apply the concepts, techniques, and tools learned throughout the course to real-world data science projects.
- Approach: Each project will include a problem statement, data collection, exploratory data analysis, modeling, and presentation of results.
11.2 Project Phases
- Project Selection
- Identify a real-world problem or opportunity in data science.
- Develop a clear problem statement and objectives for the project.
- Data Collection
- Gather relevant datasets from various sources (public datasets, APIs, web scraping).
- Ensure data quality and appropriateness for the problem.
- Exploratory Data Analysis (EDA)
- Conduct EDA to understand data distributions, relationships, and patterns.
- Use visualizations to communicate findings and insights.
- Data Preprocessing
- Clean and preprocess the data (handling missing values, outlier detection, feature engineering).
- Perform necessary transformations (scaling, encoding categorical variables).
- Model Development
- Choose appropriate models based on the problem type (supervised, unsupervised, time series).
- Implement multiple models and evaluate their performance using suitable metrics.
- Optimize model parameters through techniques like grid search or random search.
- Model Evaluation and Selection
- Compare models based on performance metrics (accuracy, precision, recall, F1-score).
- Select the best-performing model for deployment.
- Deployment and Presentation
- Deploy the model using a simple web application (e.g., Flask) or notebook interface.
- Create a comprehensive report summarizing the project, methodologies used, challenges faced, and key findings.
- Prepare a presentation to share results with peers, focusing on storytelling and visualization techniques.
11.3 Types of Projects
- Data Analysis Project: Analyze a dataset to derive insights and visualizations (e.g., customer segmentation, sales analysis).
- Predictive Modeling Project: Build a predictive model to forecast outcomes (e.g., housing price prediction, churn prediction).
- Time Series Project: Analyze and forecast time series data (e.g., stock prices, sales forecasting).
- NLP Project: Create a model for text classification, sentiment analysis, or language generation (e.g., news categorization, chatbot).
- Computer Vision Project: Develop an image classification or object detection model (e.g., identifying plant species, facial recognition).
- Generative Models Project: Use GANs to create synthetic data or images (e.g., generating artwork or realistic images).
11.4 Final Showcase
- Conduct a final showcase where students present their projects to the class.
- Engage in Q&A sessions to discuss methodologies, results, and potential improvements.
- Encourage peer feedback and collaboration on project ideas.